Dumper helper
1Overview
This helper is used to write the contents of a variable, in a more usable style than PHP's native print_r() and var_dump() functions.
It can generate a stream in plain text, ANSI-formatted text or HTML.
To display the contents of a variable in a Smarty template, you can use the dedicated Smarty modifier and block tag.
The static methods dumpText(), dumpAnsi() and dumpHtml() are used to generate a dump directly from a variable. The static die() method is used to write a dump from a variable and then exit.
2Example data
Here's the data used in the following examples:
class Foo {
protected int $bb = 12;
public string $aa = 'abcdef';
static public float $cc = 12.3;
}
$foo = new Foo();
$var = [
1,
'bar' => 2.34,
[
'baz',
[123, 'fubar', 'qux'],
],
"abc\ndef\nghi",
$foo,
];
3die(): dump and stop processing
The static die() method writes the contents of a variable (adapted to the environment, like the dump() method below), then stops processing (call exit(1)).
Example of use:
use \Temma\Utils\Dumper as TµDumper;
TµDumper::die($var);
4dump(): adaptive generation
The static dump() method detects whether the code is running on the command line or in a web environment. Accordingly, it calls the dumpAnsi() or dumpHtml() method (see these methods below).
Example of use:
use \Temma\Utils\Dumper as TµDumper;
$text = TµDumper::dump($var);
print($text);
5dumpText(): plain text generation
Example of use:
$text = \Temma\Utils\Dumper::dumpText($var);
print($text);
Resultat:
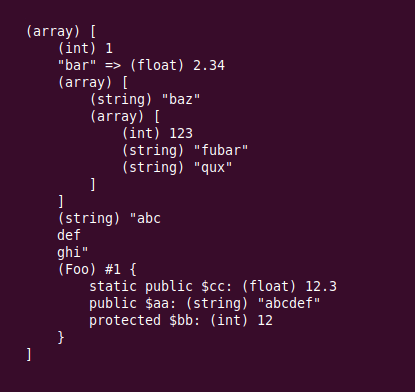
6dumpAnsi(): ANSI text generation
Example of use:
$text = \Temma\Utils\Dumper::dumpAnsi($var);
print($text);
Resultat:
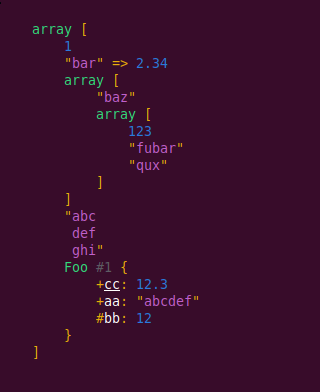
7dumpHtml(): HTML generation
Example of use:
$text = \Temma\Utils\Dumper::dumpHtml($var);
print($text);
Resultat:
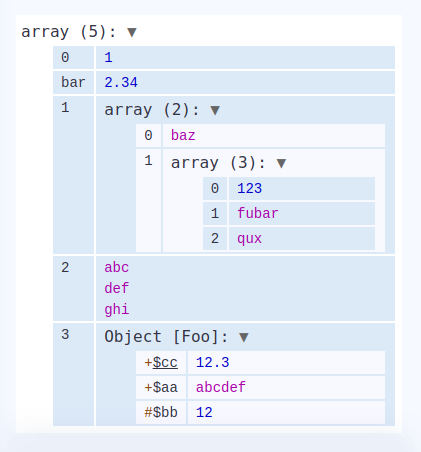