Execution flow
1Presentation
It is possible to request the execution of plugins, before and/or after the execution of the application controllers. Technically, plugins are controllers with special methods, which can modify the request before passing it on to the next plugin or to the designated controller. You can chain plugins before the controller, and other plugins after.
The source files for plugins are stored in the controllers directory of the application.
The same object can have both plugin and controller roles.
2Execution control
Temma's execution chain has 3 main phases:
- The pre-plugins are executed one after the other.
-
In the controller:
- The __wakeup() initialization method is executed.
-
The action is executed. This may be:
- The __invoke() proxy action if defined.
- The requested action based on the URL, if defined.
- The __call() default action if defined.
- The __sleep() finalization method is executed.
- The post-plugins are executed one after the other.
Each of these methods can influence the flow of execution. By default, if they return nothing (or if they return null or self::EXEC_FORWARD, see below), execution proceeds to the next step. Otherwise, they can return different values, to influence the flow of execution by going back or by skipping steps.
Here is a summary diagram, with the different possible execution flows:
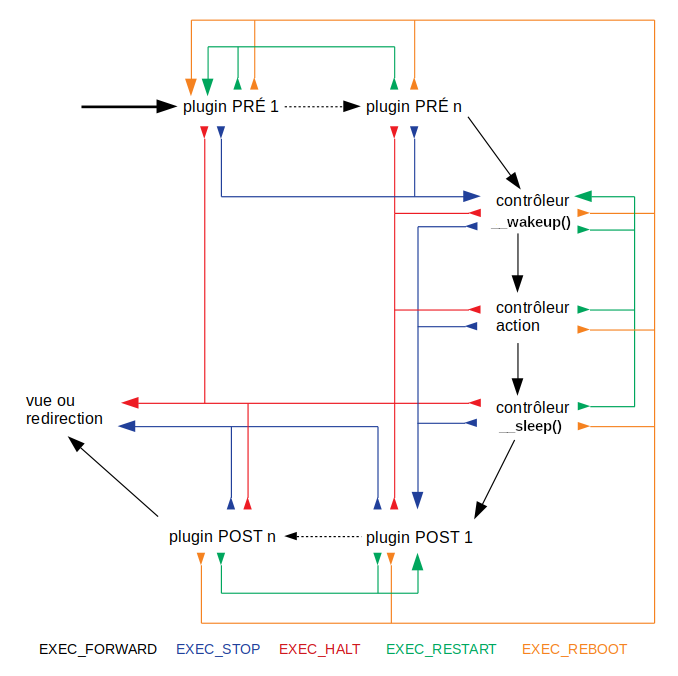
2.1EXEC_FORWARD
Goes to the next step of the execution chain.
The method must return:
// no return
return null;
return self::EXEC_FORWARD;
return \Temma\Web\Controller::EXEC_FORWARD;
It is also possible to throw an exception (in the action or in a function called by it):
throw new \Temma\Exceptions\FlowForward();
2.2EXEC_STOP
Stops the execution of the current phase (pre-plugins, controller or post-plugins):
- By a pre-plugin: no other pre-plugin will be executed and we will go directly to the controller phase.
- By the controller initialization method: neither the action nor the finalization method will be executed, and we will go directly to the post-plugins phase.
- By the action of the controller: the finalization method will not be executed and we will go directly to the post-plugins phase.
- By the controller finalization method: we will go to the post-plugins phase (as with EXEC_FORWARD).
- By a post-plugin: no other post-plugin will be executed.
The method must return:
return self::EXEC_STOP;
return \Temma\Web\Controller::EXEC_STOP;
It is also possible to throw an exception (in the action or in a function called by it):
throw new \Temma\Exceptions\FlowStop();
2.3EXEC_HALT
Stops the entire execution chain and goes directly to the view.
- By a pre-plugin: no other pre-plugin will be executed, neither the controller phase, nor the post-plugin phase.
- By the controller initialization method: neither the action nor the finalization method will be executed, as will the post-plugin phase.
- By the action of the controller: the finalization method will not be executed, as will the post-plugin phase.
- By the controller finalization method: the post-plugin phase will not be executed.
- By a post-plugin: no other post-plugin will be executed.
The method should return:
return self::EXEC_HALT;
return \Temma\Web\Controller::EXEC_HALT;
It is also possible to throw an exception (in the action or in a function called by it):
throw new \Temma\Exceptions\FlowHalt();
2.4EXEC_RESTART
Restarts the processing of the current phase (pre-plugins, controller or post-plugins).
- By a pre-plugin: the whole chain of pre-plugins will be executed again.
- By the controller initialization method: The initialization method will be executed again.
- By the controller action: the initialization method will be executed again.
- By the controller finalization method: the initialization method will be executed again.
- By a post-plugin: the whole chain of post-plugins will be executed again.
The method should return:
return self::EXEC_RESTART;
return \Temma\Web\Controller::EXEC_RESTART;
It is also possible to throw an exception (in the action or in a function called by it):
throw new \Temma\Exceptions\FlowRestart();
2.5EXEC_REBOOT
Restarts the entire processing chain (pre-plugins + controller + post-plugins). Regardless of the location, execution resumes at the first pre-plugin and unwinds execution.
The method should return:
return self::EXEC_REBOOT;
return \Temma\Web\Controller::EXEC_REBOOT;
It is also possible to throw an exception (in the action or in a function called by it):
throw new \Temma\Exceptions\FlowReboot();
2.6EXEC_QUIT
Stops the execution of the framework. From a plugin or a controller, this interrupts all processing; neither other plugins, nor the controller, will be executed. Unlike EXEC_HALT, the view will not be executed and redirection requests are ignored.
The method should return:
return self::EXEC_QUIT;
return \Temma\Web\Controller::EXEC_QUIT;
It is also possible to throw an exception (in the action or in a function called by it):
throw new \Temma\Exceptions\FlowQuit();