Command-line interface
1Presentation
It is often useful to write scripts to be executed from the command line. This is very easy to do in pure PHP, but you then have to manually initialize the log, data sources, autoloader, dependency injection...
Temma therefore offers the Comma (COMmand-line MAnager) feature, which performs all the necessary initializations before executing the requested code.
A script is executed by calling the bin/comma program, passing as parameters
the name of the object and method to be executed, as well as any additional parameters expected by this method.
The code that executes can either be completely autonomous (doing its job based on the options provided),
or enter into interactive communication with the user.
Some imaginary examples:
New data pushed to CRM
Please enter the path to the file to import: /tmp/import.json
Import complete
By default, executable commands are stored in the project's cli/ directory. However, you can place them wherever you like in the include paths, possibly using a namespace.
When using a namespace, you must enclose the object name in quotes.
For example:
New data pushed to CRM
Please enter the path to the file to import: /tmp/import.json
Import complete
Commands provided by Temma
Temma provides a number of commands, the documentation for which can be found in the "Helpers" section:
- \Temma\Cli\Temma: To update the framework source code.
- \Temma\Cli\Cache: To clear the cache.
- \Temma\Cli\User: To manage users (compatible with the Auth plugin/controller, the Auth attribute and the Api plugin).
2Documentation
By typing bin/comma or bin/comma help, you can view the general Comma documentation:
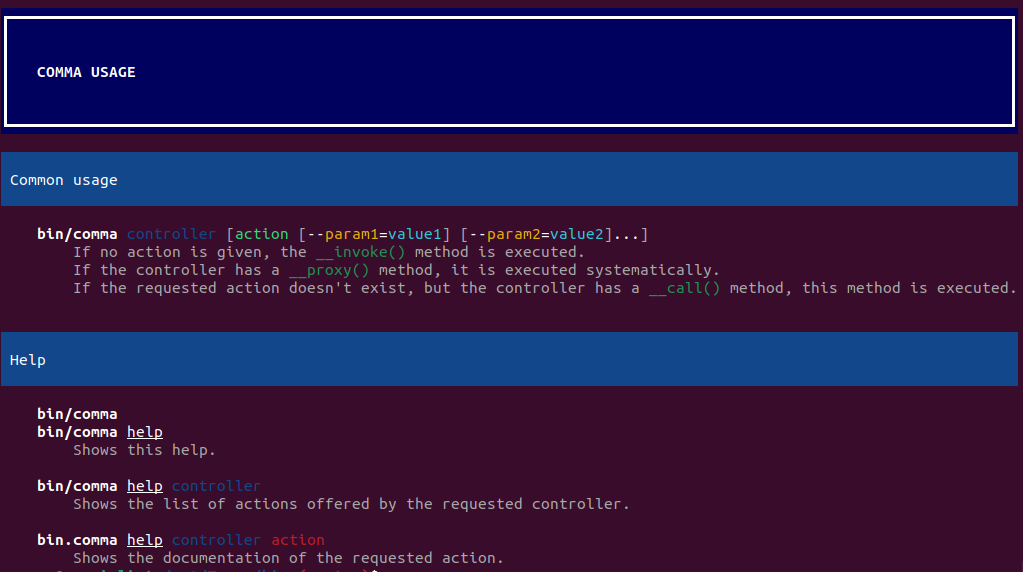
If you type bin/comma help followed by the name of a controller, you'll see a list of the controller's actions, with associated documentation:
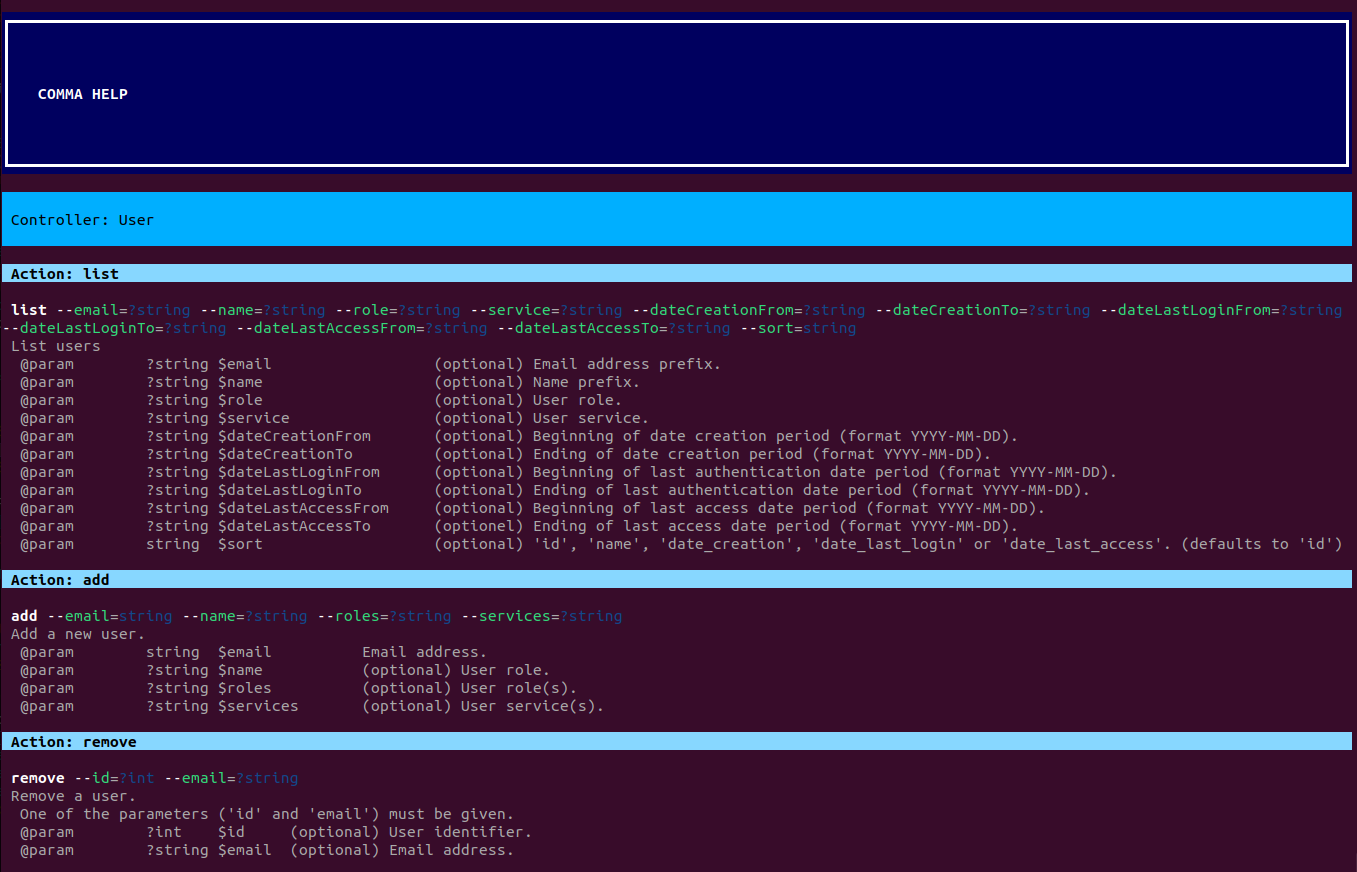
If you add the name of an action, you will only see its documentation:
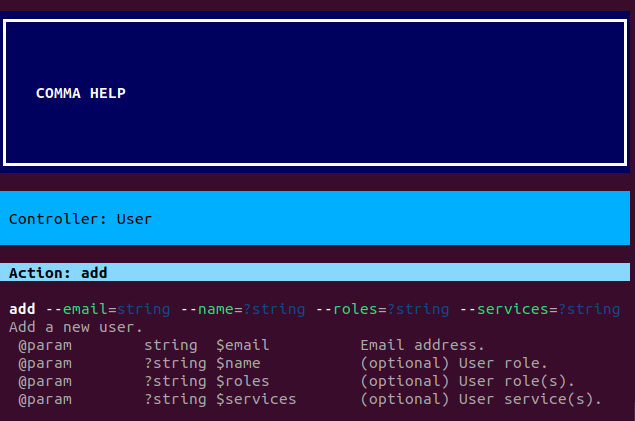
3Controllers
The objects executed by Comma are controllers identical to those used by Temma in response to HTTP requests, except that they must be stored in the cli/ directory (and not controllers/).
There are many similarities:
- Actions can take a variable number of parameters, with optional parameters (having a default value).
- The __wakeup() initialization and __sleep() finalization methods are executed before and after the action execution respectively.
- If no method name is provided on the command line, the __invoke() root action is called. This action cannot take parameters.
- If a __call() default action is defined in the controller, it will be executed for all requested actions that do not have an equivalent method.
- If a __proxy() proxy action is defined in the controller, it will always be executed, regardless of the action called.
- Attributes set on controllers and actions are executed (provided they can run in a command line environment, not a web environment).
- The etc/temma.php configuration file is read and its contents made available via the $this->_config object.
- The dependency injection component is generated, and is available in the same way (by writing $this->_loader).
- Data sources are generated, and are available either by direct writing (e.g. $this->db), or by passing through the dependency injection component (e.g. $this->_loader->dataSources->db or $this->_loader->dataSources['db-read']).
- DAOs can also be used.
But there are also several differences:
- There is no routing system, even simplified (it is not possible to call a controller by a name other than its own).
- No plugin execution.
- There are no views.
- The $this->_request object contains only the parameters supplied on the command line.
4Parameters
Parameters expected by actions are passed on the command line by adding the -- prefix, then the equal (=) sign followed by the associated value.
If the value contains spaces, it can be enclosed in quotation marks (") or apostrophes (').
If a parameter is passed without a value, it will be processed as a boolean with true value.
5Example
For example, you could create a script to add a user to the database.
This script could be called as follows:
We could imagine an optional parameter, to say that the user is an administrator:
In concrete terms, Comma will instantiate the User object, then call its add() method, passing it the parameters.
The User object must be stored in a file named User.php,
located in the cli/ directory of the project.
This object must inherit from the \Temma\Web\Controller object.
The code for this object could be:
class User extends \Temma\Web\Controller {
/** Creation of an automatic Data Access Object. */
protected $_temmaAutoDao = true;
/**
* Add user.
* @param string $name Name of the user.
* @param string $email Email address of the user.
* @param bool $admin (optional) Administrator rights. False by default.
*/
public function add(string $name, string $email, bool $admin=false) {
// add user to database
$id = $this->dao->create([
'name' => $name,
'email' => $email,
'roles' => $admin ? 'admin' : '',
]);
// message
print("User created with identifier '$id'.\n");
}
}
6Configuration
By default, command line scripts load the configuration present in the etc/temma.php file. It is possible to provide the path to another file using the conf parameter.
This parameter must be placed before the controller name.
For example:
Note that providing a file outside the project tree is possible, but will not change the paths used to the various directories. For example, the Language plugin will continue to look for its files in etc/lang/.
7Include paths
Scripts executed with Comma have the project's lib/ directory added to their include paths. Additional paths can be added with the inc parameter.
As with the conf parameter, this parameter must be placed before the controller name.
For example:
8Log
By default, command-line scripts write their logs to the error output.
If provided for in the etc/temma.php file, logs can also be written to the log/temma.log file.
To disable writing to error output, use the nostderr parameter.
As with the conf and inc parameters, this parameter must be placed before the controller name.
Example:
9Interface
The user interface is completely different from that of web controllers. Command-line scripts interact by writing to their standard output, and retrieve what the user enters by reading from their standard input.
Temma provides two helpers that are useful in these conditions:
- \Temma\Utils\Ansi: provides methods for improving the display of information written by your code.
- \Temma\Utils\Term: provides functionalities to interact more easily with the terminal.